11ty second 11ty: The Render Plugin Part 1
The Render plugin is comprised of two shortcodes for use in your Nunjucks, Liquid or JS templates. It’s a plugin that is bundled with the main 11ty NPM package and ready to use as soon as you nom install 11ty.
To get started with the plugin, you need to install it in your .eleventy.js
config file by requiring the plugin and then initializing it
const { EleventyRenderPlugin } = require("@11ty/eleventy");
module.exports = function(eleventyConfig) {
eleventyConfig.addPlugin(EleventyRenderPlugin);
}
The two shortcodes it adds to your project are renderTemplate
and renderFile
.
In this part, we’ll tackle renderTemplate
.
renderTemplate
allows you to put a string between two matching shortcodes and render that string in a templating language different from that of the current template
Using the renderTemplate
tag
Let's take a quick look at the syntax for the tag.
<!-- In Nunjucks -->
{% renderTemplate <template-string> <optional-data> %}
<!-- In Liquid -->
{% renderTemplate <template-string>, <optional-data> %}
renderTemplate
arguments
The renderTemplate tag accepts two arguments: at templating language string, and an optional data variable.
Template language argument
The template language argument accepts any template string that eleventyConfig accepts. The current list of languages are html,liquid,ejs,md,hbs,mustache,haml,pug,njk,11ty.js
. Multiple template languages can be chained together. For instance, if you want to process Liquid tags and then Markdown, you can use the string liquid,md
to interpret the template in that order.
Data argument (optional)
The second argument for the tag is the data argument. While you have access to the eleventy
and page
data by default, any additional data needs to be explicitly passed in. This includes various data files as well as frontmatter from the current page or template.
Using Liquid filters in a Nunjucks template
<!-- ...head, etc. -->
<body>
<p>The date is: {{ settings.today }}</p>
</body>
Here we have a Nunjucks index page. In this page, we have a date time. This is being generated by a global JS data file named settings.js
in my _data
directory.
It's a JavaScript datetime string. It’s not great to read for a human.

I want to reformat that in the template with a filter, but uh-oh, Nunjucks doesn’t have a date filter! Now that my project has the renderTemplate paired shortcode, I can get to work.
<body>
{% renderTemplate "liquid", settings %}
<p>The date is: {{ today | date: "%B %d, %y" }}</p>
{% endrenderTemplate %}
</body>
To start, we'll tell the tag to use Liquid to parse the string, and we'll pass in the settings
data from the global data file.
Anything between the renderTemplate
and the endRenderTemplate
will be rendered in Liquid instead of Nunjucks and have access to the global data.
The Liquid filter has a powerful set of controls that let me reformat the date into something much nicer.

Or for a simpler use case, sometimes writing Markdown is more ergonomic than writing HTML, so why not embed a little Markdown in your HTML
{% renderTemplate "liquid,md" %}
# I am a title
* I am a list
* I am a list
1. I am an ordered list
1. i'm actually second in an ordered list test
{% endrenderTemplate %}
This Markdown will generate a full set of HTML just like a .md
file would.
<h1>I am a title</h1>
<ul>
<li>I am a list</li>
<li>I am a list</li>
</ul>
<ol>
<li>I am an ordered list</li>
<li>i'm actually second in an ordered list test</li>
</ol>
Template engines all the way down!
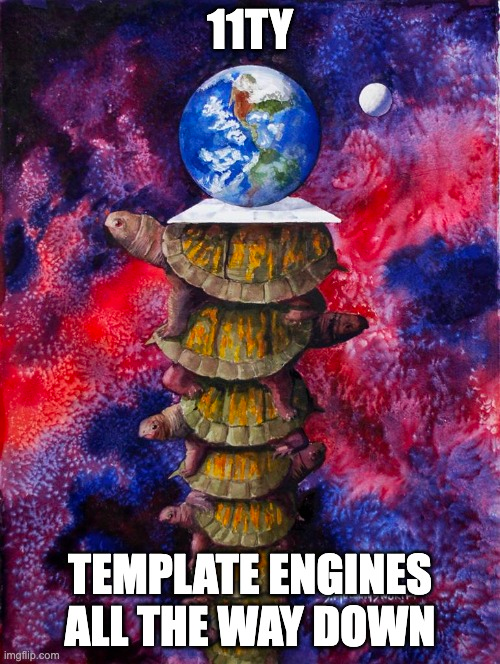
This plugin opens the door to a lot of new possibilities. Any templating language can be embedded in any page or template! 11ty: It's templates all the way down!
Just want the code? Check the 11ty Second 11ty repo for this and others!